Return to sender
You can save yourself a lot of headache by being careful with what and how you return from functions and methods.
Returning thingsโ
This post is about returning things. I'd like to talk about it in the context of data access, since that's something you'll be working with a lot. It applies to anytime you are returning something though.
What you return from a function or method has a lot of implications for how your code looks upstream.
Imagine you're working with Mongoose and MongoDB and you want to get a user. You might have something like this:
const getUserByEmail = async (email) => {
try {
const user = await UserModel.findOne({ email: email });
return user;
} catch (error) {
// Do something with error
}
};
All well and good, but what does Mongoose do when it can't find something? From the docs
The result of the query is a single document, or null if no document was found.
OK well then, we now have the possibility of returning null
, which means we have an inconsistent return type for our getUserByEmail
function. This wouldn't fly in a strongly typed language, but here we are with JavaScript.
If we use this function as is we have just condemned ourselves to forever doing defensive checks when we use the function:
if (getUserByEmail !== null) {
// Do cool things with the user
}
Make it betterโ
What a pain! We can make our life easier and our code cleaner by simply ensuring that our function always returns a user:
const getUserByEmail = async (email) => {
try {
const user = await UserModel.findOne({ email: email });
if (user === null) {
throw new Error("User not found");
}
return user;
} catch (error) {
// Do something with error
}
};
By doing our defensive check here at the source of truth we don't have to to that defensive check upstream. Remember, you define how your code behaves:
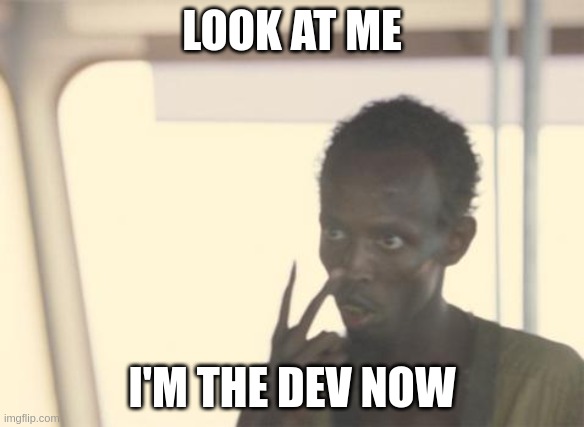
I'm a real dev now!
So make things easy on yourself, think carefully about what you are returning and you will write better code ๐